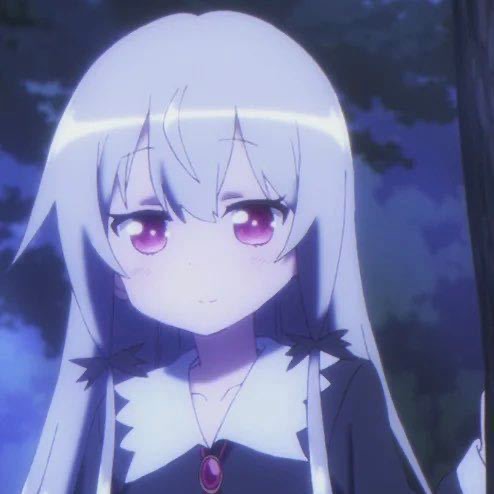
01-复杂度1 最大子列和问题 (20 分)
给定K个整数组成的序列{ N1, N2, …, N**K },“连续子列”被定义为{ N**i, N**i+1, …, N**j },其中 1≤i≤j≤K。“最大子列和”则被定义为所有连续子列元素的和中最大者。例如给定序列{ -2, 11, -4, 13, -5, -2 },其连续子列{ 11, -4, 13 }有最大的和20。现要求你编写程序,计算给定整数序列的最大子列和。
本题旨在测试各种不同的算法在各种数据情况下的表现。各组测试数据特点如下:
- 数据1:与样例等价,测试基本正确性;
- 数据2:102个随机整数;
- 数据3:103个随机整数;
- 数据4:104个随机整数;
- 数据5:105个随机整数;
输入格式:
输入第1行给出正整数K (≤100000);第2行给出K个整数,其间以空格分隔。
输出格式:
在一行中输出最大子列和。如果序列中所有整数皆为负数,则输出0。
输入样例:
1 | 6 |
输出样例:
1 | 20 |
解法一(暴力):
1 | /*思路:运用两层循环,第一层循环用来确定子列的第一个元素,第二层循环用来遍历每个已经确定了第一个元素的子列 |
解法二(递归):
1 | //这是一种分而治之的思想 |
解法三(在线处理):
1 | int MaxSubseqSum(int A[], int N){ |
01-复杂度2 Maximum Subsequence Sum (25 分)
Given a sequence of K integers { N1, N2, …, N**K }. A continuous subsequence is defined to be { N**i, N**i+1, …, N**j } where 1≤i≤j≤K. The Maximum Subsequence is the continuous subsequence which has the largest sum of its elements. For example, given sequence { -2, 11, -4, 13, -5, -2 }, its maximum subsequence is { 11, -4, 13 } with the largest sum being 20.
Now you are supposed to find the largest sum, together with the first and the last numbers of the maximum subsequence.
Input Specification:
Each input file contains one test case. Each case occupies two lines. The first line contains a positive integer K (≤10000). The second line contains K numbers, separated by a space.
Output Specification:
For each test case, output in one line the largest sum, together with the first and the last numbers of the maximum subsequence. The numbers must be separated by one space, but there must be no extra space at the end of a line. In case that the maximum subsequence is not unique, output the one with the smallest indices i and j (as shown by the sample case). If all the K numbers are negative, then its maximum sum is defined to be 0, and you are supposed to output the first and the last numbers of the whole sequence.
Sample Input:
1 | 10 |
Sample Output:
1 | 10 1 4 |
题解:
由于这题没看清题目(英语蒟蒻),导致改了半天也没AC,都快WA到吐了,就上CSDN搜了一下,原来要输出的是最大子列的首尾对应元素而不是下标。
1 |
|
未完待续…
- 本文标题:浙江大学数据结构2021春--编程题
- 本文作者:recovxy
- 创建时间:2021-04-04 12:34:54
- 本文链接:https://naiv.xyz/2021/04/04/2021-04-04-Zhejiang University Data Structure Problem Solution--Programming Problem/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!